深入解析LangChain及其应用场景
- 2569字
- 13分钟
- 2024-06-26
LangChain 是一个用于构建语言模型驱动的应用程序的框架。它提供了一系列工具和抽象层,使开发者能够更容易地集成和使用大型语言模型(LLM),如OpenAI的GPT-4或其他类似的模型。以下是对LangChain的详细解析以及它与LLM之间的联系和应用场景。
什么是LangChain?
抽象与简化
LangChain 提供了一些高级抽象和工具,帮助开发者简化与LLM交互的过程。这包括对模型的调用、数据的预处理和后处理、上下文管理、状态追踪等。
模块化设计
LangChain的设计是模块化的,允许开发者根据具体需求选择和组合不同的组件。例如,输入输出处理模块、内存管理模块、模型交互模块等。
集成能力
它可以与各种数据源和外部API集成,方便地获取和处理外部数据,从而扩展LLM的功能。
LangChain与LLM的联系
依赖关系
LangChain 本质上是围绕LLM构建的框架。它依赖于LLM来执行核心的自然语言处理任务,如文本生成、问题回答、翻译等。
增强功能
虽然LLM本身非常强大,但LangChain通过提供额外的工具和抽象层,增强了LLM的可用性和适用性。例如,它可以帮助管理长上下文、处理多轮对话、维护会话状态等。
LangChain的独特性
简化开发流程
LangChain通过其简化的接口和工具,使开发者能够更容易地构建和调试LLM驱动的应用程序。这减少了开发的复杂性和时间成本。
功能扩展
它提供了一些LLM本身不具备的功能,例如长上下文处理、复杂任务的分解与协调、多模型协同工作等。
社区支持
由于LangChain有一个活跃的社区和不断更新的文档,开发者可以从中受益,获得最新的最佳实践和技术支持。
LangChain的应用场景
虽然许多LLM驱动的应用程序可以通过直接调用LLM API来实现,但在以下场景中,LangChain的优势尤为明显:
需要复杂上下文管理的对话系统
LangChain的上下文管理工具使得处理复杂、多轮对话变得更加容易和高效。
多数据源集成
LangChain可以无缝集成多种外部数据源和API,这在需要从多个来源获取数据并进行处理的应用中非常有用。
高度定制化的任务
当任务需要高度定制化的输入输出处理和逻辑控制时,LangChain提供的模块化设计和扩展能力能够满足这些需求。
需要快速开发和迭代的项目
LangChain提供的高层次抽象和工具能够加速开发过程,适合需要快速迭代和原型开发的项目。
LangChain的应用场景实例
示例1:复杂上下文管理的对话系统
以下是一个使用LangChain构建复杂上下文管理对话系统的Node.js示例:
1const { LangChain } = require("langchain");2
3// 初始化LangChain4const chain = new LangChain({5 model: "gpt-4",6 apiKey: "your-api-key",7});8
9// 定义上下文管理器10const contextManager = chain.createContextManager();11
12// 添加初始上下文13contextManager.addContext("user", "Hello, how can I help you today?");14
15// 处理用户输入并生成响应16async function handleUserInput(input) {17 contextManager.addContext("user", input);18 const response = await chain.generateResponse(contextManager.getContext());19 contextManager.addContext("bot", response);20 return response;21}22
23// 示例用户输入24handleUserInput("I need help with my order.").then((response) => {25 console.log("Bot:", response);26});
解释:
LangChain
:这是LangChain的核心类,用于初始化并配置语言模型。contextManager
:用于管理对话的上下文。addContext
方法用于添加新的上下文信息。handleUserInput
:这是一个处理用户输入并生成响应的异步函数。它将用户输入添加到上下文中,然后生成响应并将响应添加到上下文中。
参数示例:
- 用户输入:
'I need help with my order.'
- 生成的响应示例:
'Sure, I can help you with your order. What seems to be the problem?'
示例2:多数据源集成
以下是一个使用LangChain集成多个数据源的Node.js示例:
1const { LangChain } = require("langchain");2const axios = require("axios");3
4// 初始化LangChain5const chain = new LangChain({6 model: "gpt-4",7 apiKey: "your-api-key",8});9
10// 定义数据源获取函数11async function getDataFromSource1() {12 const response = await axios.get("https://api.source1.com/data");13 return response.data;14}15
16async function getDataFromSource2() {17 const response = await axios.get("https://api.source2.com/data");18 return response.data;19}20
21// 集成多个数据源并生成响应22async function generateResponse() {23 const data1 = await getDataFromSource1();24 const data2 = await getDataFromSource2();25
26 // 组合数据27 const combinedData = {28 source1: data1,29 source2: data2,30 };31
32 const response = await chain.generateResponse(combinedData);33 return response;34}35
36// 示例调用37generateResponse().then((response) => {38 console.log("Generated Response:", response);39});
解释:
getDataFromSource1
和getDataFromSource2
:分别从两个不同的数据源获取数据。combinedData
:将两个数据源的数据组合成一个对象,source1
和source2
是对象的键,用于标识不同的数据源。generateResponse
:将组合的数据传递给LangChain以生成响应。
参数示例:
data1
示例:{ "name": "John", "order": "12345" }
data2
示例:{ "status": "shipped", "deliveryDate": "2024-06-30" }
- 生成的响应示例:
'Order 12345 for John has been shipped and will be delivered by 2024-06-30.'
示例3:高度定制化的任务
以下是一个使用LangChain处理高度定制化任务的Node.js示例:
1const { LangChain } = require("langchain");2
3// 初始化LangChain4const chain = new LangChain({5 model: "gpt-4",6 apiKey: "your-api-key",7});8
9// 定义自定义输入输出处理函数10function customInputProcessor(input) {11 return `Processed input: ${input}`;12}13
14function customOutputProcessor(output) {15 return `Processed output: ${output}`;16}17
18// 处理自定义任务19async function handleCustomTask(input) {20 const processedInput = customInputProcessor(input);21 const response = await chain.generateResponse(processedInput);22 const processedOutput = customOutputProcessor(response);23 return processedOutput;24}25
26// 示例调用27handleCustomTask("Custom task input").then((response) => {28 console.log("Custom Task Response:", response);29});
解释:
customInputProcessor
:自定义的输入处理函数,将输入进行处理。customOutputProcessor
:自定义的输出处理函数,将输出进行处理。handleCustomTask
:处理自定义任务的异步函数,使用自定义的输入和输出处理函数。
参数示例:
- 输入示例:
'Custom task input'
- 处理后的输入示例:
'Processed input: Custom task input'
- 生成的响应示例:
'This is a response to the processed input.'
- 处理后的输出示例:
'Processed output: This is a response to the processed input.'
示例4:使用模板生成响应
LangChain允许使用模板来生成响应,这对于需要根据特定格式生成文本的应用非常有用。以下是一个示例:
1const { LangChain } = require("langchain");2
3// 初始化LangChain4const chain = new LangChain({5 model: "gpt-4",6 apiKey: "your-api-key",7});8
9// 定义模板10const template = `11 Dear {{name}},12
13 Thank you for reaching out to us regarding {{issue}}. We are currently looking into it and will get back to you shortly.14
15 Best regards,16 Support Team17`;18
19// 使用模板生成响应20async function generateResponseWithTemplate(data) {21 const response = await chain.generateResponse(template, data);22 return response;23}24
25// 示例调用26generateResponseWithTemplate({ name: "John Doe", issue: "your order" }).then(27 (response) => {28 console.log("Generated Response:", response);29 },30);
解释:
template
:定义了一个包含占位符的模板字符串,占位符用{{ }}
包裹。generateResponseWithTemplate
:使用模板和数据生成响应的异步函数,将数据填充到模板中。
参数示例:
- 输入数据示例:
{ name: 'John Doe', issue: 'your order' }
- 生成的响应示例:
1 Dear John Doe,2
3 Thank you for reaching out to us regarding your order. We are currently looking into it and will get back to you shortly.4
5 Best regards,6 Support Team
示例5:链式调用
LangChain支持链式调用,允许将多个操作组合在一起执行。以下是一个示例:
1const { LangChain } = require("langchain");2
3// 初始化LangChain4const chain = new LangChain({5 model: "gpt-4",6 apiKey: "your-api-key",7});8
9// 定义链式调用10async function chainOperations(input) {11 // 第一步:翻译为法语12 const step1 = await chain.generateResponse(`Translate to French: ${input}`);13
14 // 第二步:总结法语文本15 const step2 = await chain.generateResponse(`Summarize: ${step1}`);16
17 // 第三步:将总结的文本翻译回英语18 const step3 = await chain.generateResponse(19 `Translate back to English: ${step2}`,20 );21
22 return step3;23}24
25// 示例调用26chainOperations("This is a test sentence.").then((response) => {27 console.log("Chained Response:", response);28});
解释:
chainOperations
:这是一个链式调用的异步函数,依次执行多个步骤。- 第一步:将输入文本翻译为法语。
- 第二步:总结翻译后的法语文本。
- 第三步:将总结的法语文本翻译回英语。
参数示例:
- 输入示例:
'This is a test sentence.'
- 生成的响应示例:
'This is a summarized test sentence in English.'
示例6:使用LangChain模板和链式调用实现复杂任务
以下是一个结合模板和链式调用来实现复杂任务的Node.js示例:
1const { LangChain } = require("langchain");2
3// 初始化LangChain4const chain = new LangChain({5 model: "gpt-4",6 apiKey: "your-api-key",7});8
9// 定义模板10const template = `11 Hi {{name}},12
13 We have received your request regarding {{issue}}. Here is a summary of the information you provided:14
15 {{summary}}16
17 We will get back to you with more details shortly.18
19 Best,20 Support Team21`;22
23// 定义数据源获取和处理函数24async function getDataAndProcess(input) {25 // 数据源1:用户输入26 const userInput = input;27
28 // 数据源2:模拟的外部数据29 const externalData = await new Promise((resolve) => {30 setTimeout(() => {31 resolve("This is some external data related to the issue.");32 }, 1000);33 });34
35 // 生成总结36 const summary = await chain.generateResponse(37 `Summarize: ${userInput} and ${externalData}`,38 );39
40 return { summary };41}42
43// 使用模板和链式调用生成响应44async function generateComplexResponse(data) {45 const processedData = await getDataAndProcess(data.issue);46 const completeData = { ...data, ...processedData };47 const response = await chain.generateResponse(template, completeData);48 return response;49}50
51// 示例调用52generateComplexResponse({ name: "Jane Doe", issue: "a billing problem" }).then(53 (response) => {54 console.log("Generated Complex Response:", response);55 },56);
解释:
template
:定义了一个包含占位符的模板字符串。getDataAndProcess
:获取数据并生成总结的异步函数,模拟从外部数据源获取数据并生成总结。generateComplexResponse
:结合模板和链式调用生成复杂响应的异步函数。
参数示例:
- 输入数据示例:
{ name: 'Jane Doe', issue: 'a billing problem' }
- 生成的响应示例:
1 Hi Jane Doe,2
3 We have received your request regarding a billing problem. Here is a summary of the information you provided:4
5 This is a summary of the user input and some external data related to the issue.6
7 We will get back to you with more details shortly.8
9 Best,10 Support Team
结论
LangChain通过其强大的抽象和工具集,简化了与LLM交互的复杂性,并扩展了LLM的应用场景,使得构建复杂、智能的自然语言处理应用变得更加容易和高效。无论是处理复杂上下文管理的对话系统、集成多数据源的应用,还是处理高度定制化的任务,LangChain都能提供有效的解决方案。
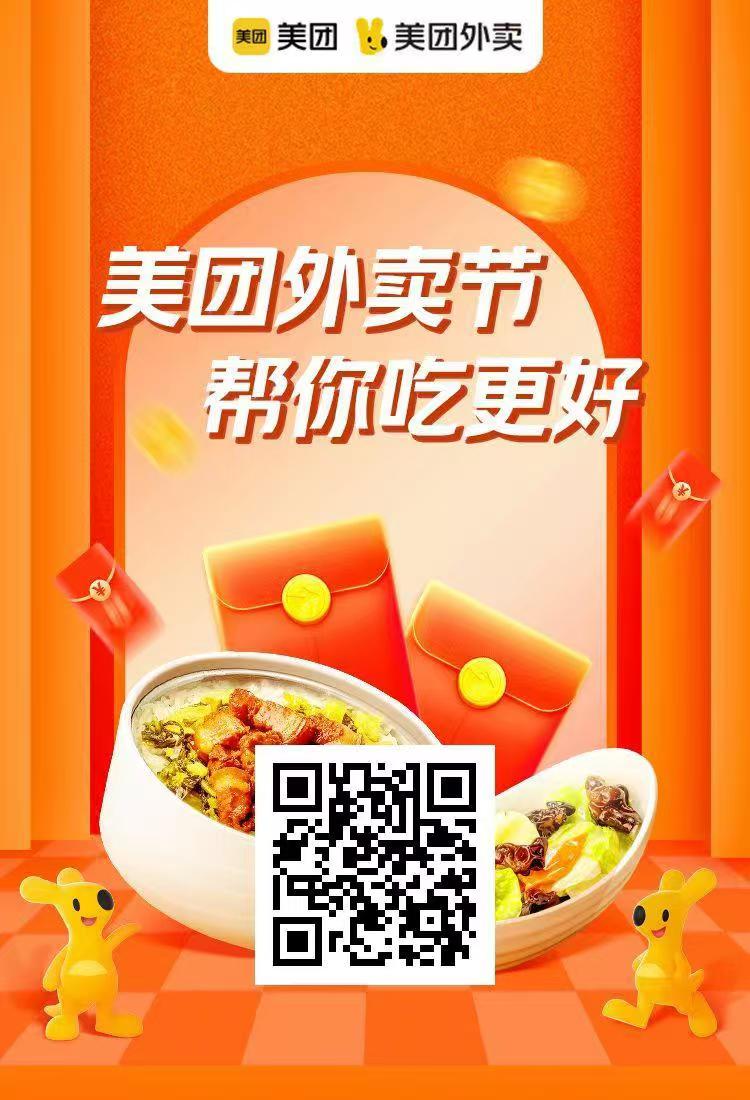
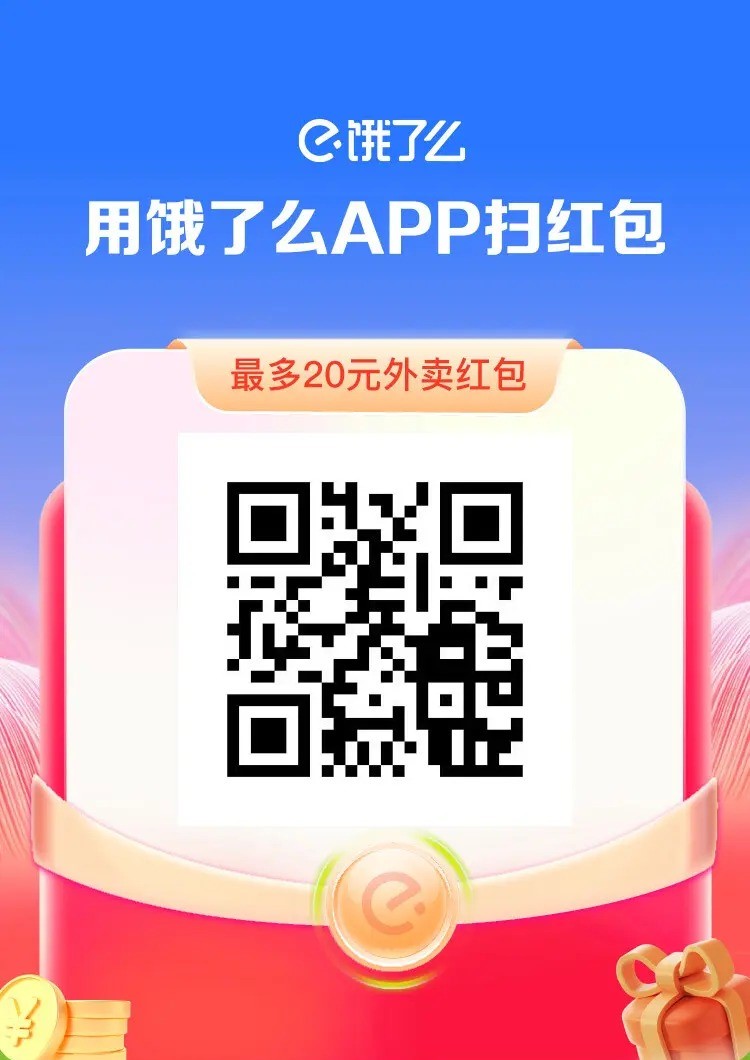
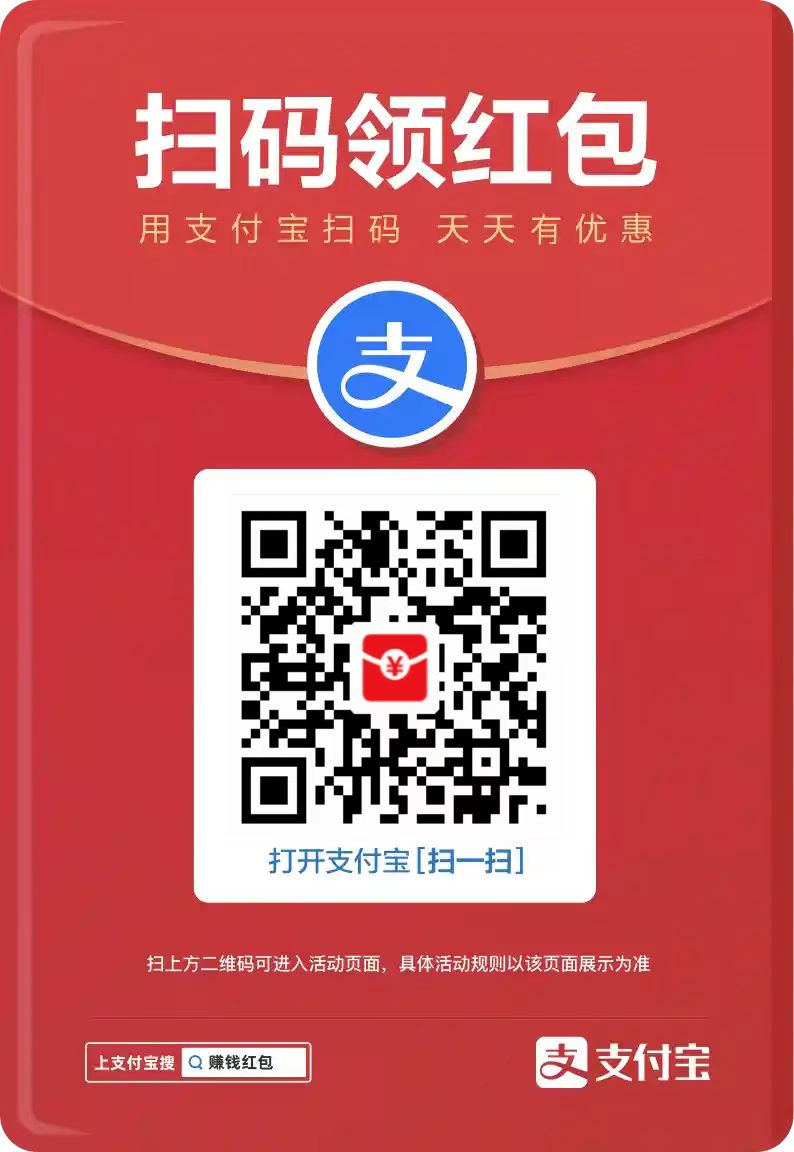