前端实现实心半圆和空心半圆的几种方式
- 828字
- 4分钟
- 2024-08-06
在前端开发中,有时候我们会遇到使用半圆的情景,比如在优惠券中,如下图所示:
本文将介绍如何使用不同的技术实现实心半圆和空心半圆。我们将覆盖三种主要的实现方式:CSS、SVG和Canvas。每种方法都包含详细的代码示例和解释。
实现实心半圆
使用 CSS
通过适当的 border-radius
设置,可以创建实心半圆。如下所示:
1<div class="square-solid-half-circle"></div>2
3<style>4 .square-solid-half-circle {5 width: 100px;6 height: 50px; /* 高度为半个圆的高度 */7 background-color: #3498db;8 border-top-left-radius: 50px;9 border-top-right-radius: 50px;10 }11</style>
解释:
width
和height
设置了宽高。background-color
设置了半圆的填充颜色。border-top-left-radius
和border-top-right-radius
设置了圆角,使其成为半圆形状。
使用 SVG
SVG 提供了精确绘制半圆的功能。以下是实现实心半圆的代码示例:
1<svg width="100" height="50">2 <path d="M 0 50 A 50 50 0 0 1 100 50" fill="#3498db" />3</svg>
解释:
path
元素中的A
指令绘制了半圆弧,fill
设置了填充颜色。
使用 Canvas
Canvas API 允许我们使用 JavaScript 动态绘制图形。以下是实心半圆的实现:
1<canvas id="solid-half-circle" width="100" height="50"></canvas>2
3<script>4 const solidCanvas = document.getElementById("solid-half-circle");5 const solidCtx = solidCanvas.getContext("2d");6
7 solidCtx.beginPath();8 solidCtx.arc(50, 50, 50, Math.PI, 0, false); // 圆心 (50,50), 半径 50, 从 π 到 0 弧度9 solidCtx.fillStyle = "#3498db"; // 设置填充颜色10 solidCtx.fill(); // 填充路径11</script>
解释:
arc
方法绘制了半圆的弧线,fillStyle
设置填充颜色,fill
方法将其填充为实心半圆。
实现空心半圆
使用 CSS
我们可以通过一个正方形设置边框颜色,同时把下边框和右边框设置为透明,然后通过旋转实现。以下是实现代码:
1<div class="square-hollow-half-circle"></div>2
3<style>4 .square-hollow-half-circle {5 width: 100px;6 height: 100px;7 border: 5px solid #3498db; /* 设置边框颜色和宽度 */8 border-radius: 100%; /* 使边框成圆形 */9 border-bottom-color: transparent; /* 隐藏底部边框 */10 border-right-color: transparent;11 box-sizing: border-box; /* 包括边框在内计算宽度和高度 */12 transform: rotate(45deg); /* 旋转 45 度 */13 }14</style>
解释:
border
设置了边框,border-radius
使其成为圆形。border-bottom-color
,border-right-color
设置为透明以隐藏边框。transform: rotate(45deg)
用于旋转。
使用 SVG
SVG 中,利用两个路径,一个大半圆和一个小半圆的遮罩来实现空心半圆:
1<svg width="100" height="50">2 <!-- 实心半圆 -->3 <path d="M 0 50 A 50 50 0 0 1 100 50 L 0 50 Z" fill="#3498db" />4 <!-- 覆盖的白色半圆 -->5 <path d="M 5 50 A 45 45 0 0 1 95 50 L 5 50 Z" fill="#ffffff" />6</svg>
解释:
- 第一个
path
元素绘制了实心半圆。 - 第二个
path
元素绘制了一个小的白色半圆,遮罩在大半圆之上。
使用 Canvas
同理,在Canvas 中,我们可以使用两个 arc
绘制外圆和内圆,实现空心半圆:
1<canvas id="canvas-hollow-half-circle-overlay" width="100" height="50"></canvas>2
3<script>4 const canvasOverlay = document.getElementById(5 "canvas-hollow-half-circle-overlay",6 );7 const ctxOverlay = canvasOverlay.getContext("2d");8
9 // 实心半圆10 ctxOverlay.beginPath();11 ctxOverlay.arc(50, 50, 50, Math.PI, 0, false);12 ctxOverlay.fillStyle = "#3498db";13 ctxOverlay.fill();14
15 // 覆盖的白色半圆16 ctxOverlay.beginPath();17 ctxOverlay.arc(50, 50, 45, Math.PI, 0, false);18 ctxOverlay.fillStyle = "#ffffff";19 ctxOverlay.fill();20</script>
解释:
- 绘制外圈的实心半圆,然后在其上绘制一个小的白色半圆,以形成空心效果。
通过以上方法,我们可以在前端实现实心和空心半圆的各种效果。
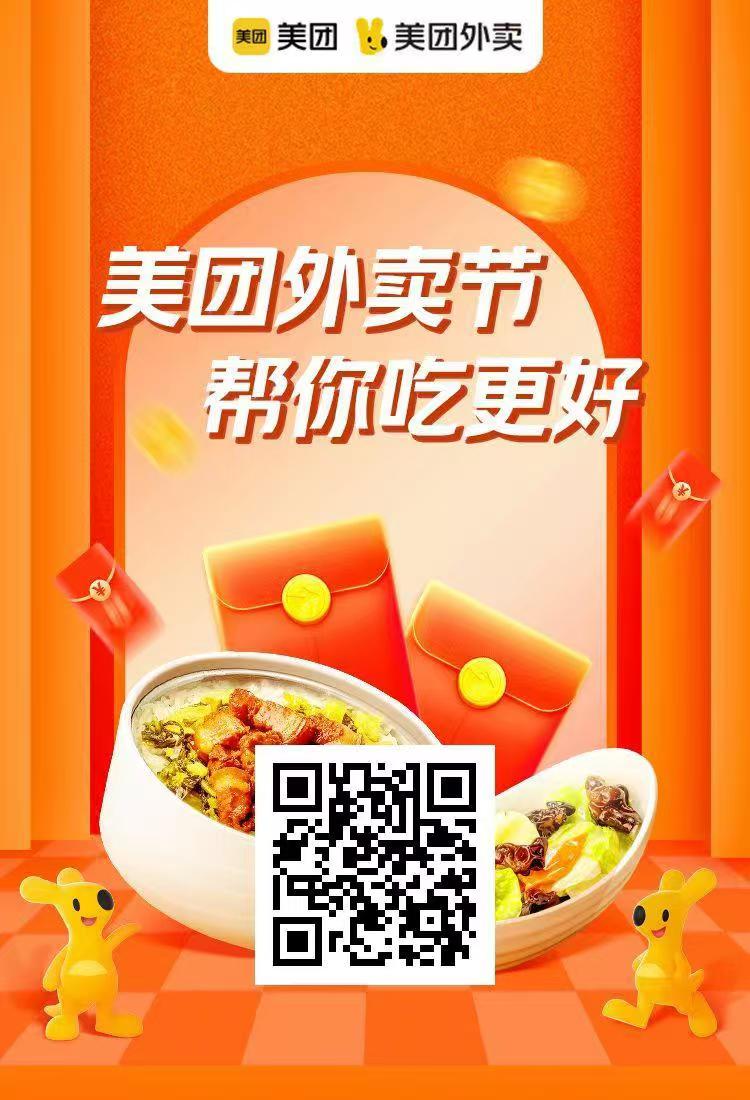
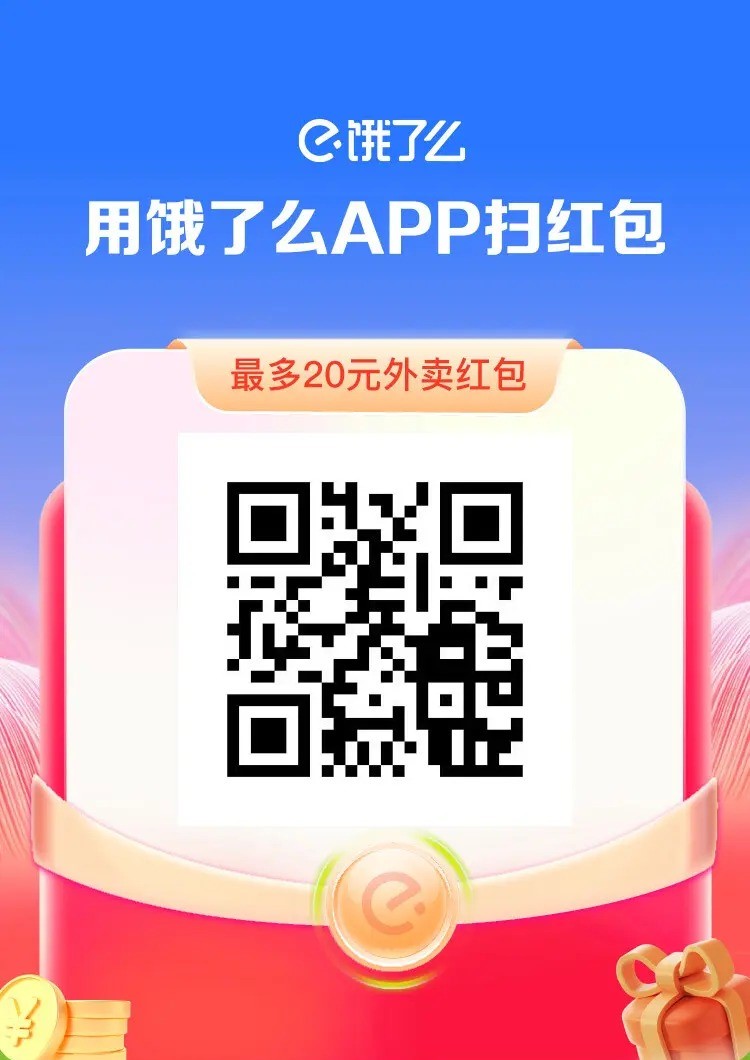
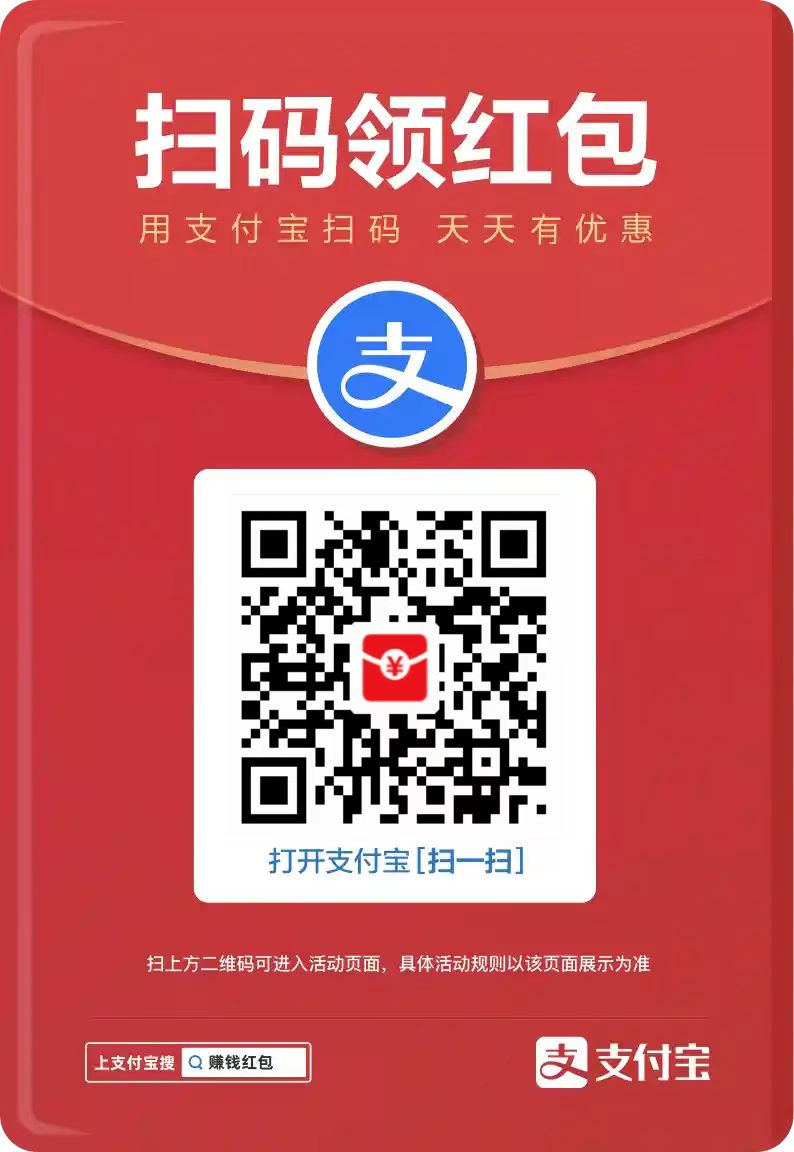